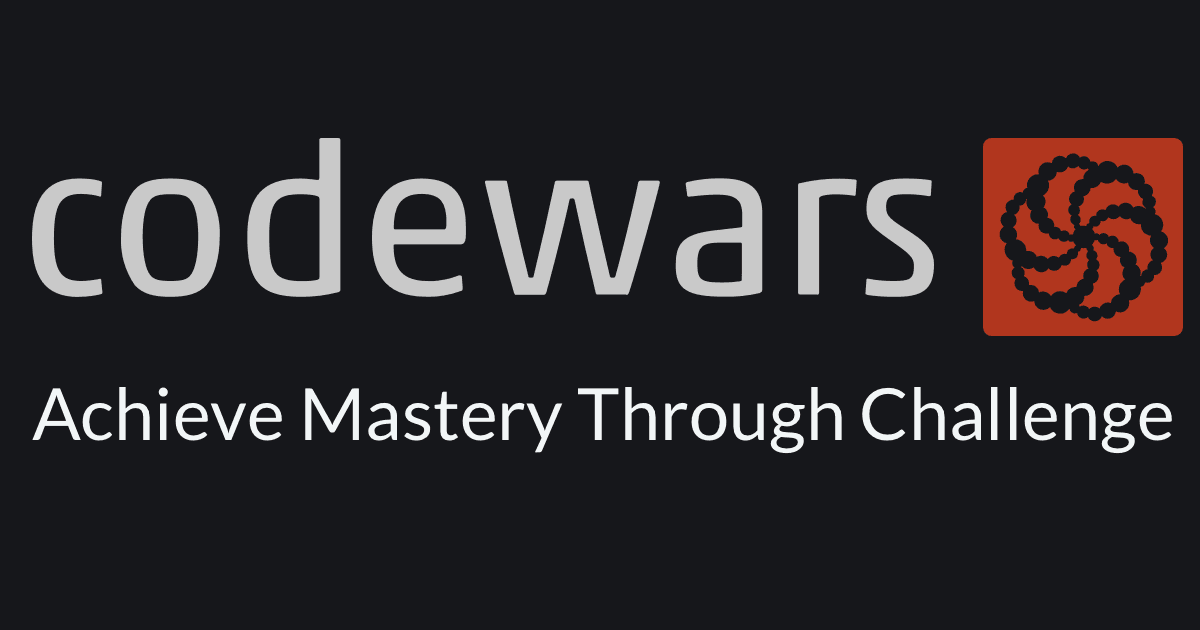
Inhalt
Die Fakten:
Plattform: | codewars.com |
Name: | Srot the inner ctonnet in dsnnieedcg oredr |
Level: | 6 kyu |
Sprache: | TypeScript |
Beschreibung:
You have to sort the inner content of every word of a string in descending order.
The inner content is the content of a word without first and the last char.
Some examples:
"sort the inner content in descending order" --> "srot the inner ctonnet in dsnnieedcg oredr"
"wait for me" --> "wiat for me"
"this kata is easy" --> "tihs ktaa is esay"
Words are made up of lowercase letters.
The string will never be null and will never be empty. In C/C++ the string is always nul-terminated.
Have fun coding it and please don't forget to vote and rank this kata! :-)
I have also created other katas. Take a look if you enjoyed this kata!
Quelle: codewars.com
Lösung
Pseudo-Code
Wie immer gibt's reichlich Varianten, hier ist eine meiner.
Erst die Lösungsschritte in Pseudo-Code. Los geht’s:
Lösungsschritte
Schritt 1
Wir loopen durch alle Wörter.
Schritt 2
Da unser Ergebnis-Array genauso lang sein wird wie das Ausgangs-Array können wir hier .map()
verwenden.
Schritt 3
Wenn ein Wort 2
oder weniger Buchstaben hat, brauchen wir es nicht verändern, da sie keinen Mittelteil haben.
Schritt 4
Wir extrahieren den mittleren Teil des aktuellen Wortes und sortieren ihn.
Schritt 5
Wir setzen das neue Wort zusammen und geben es ans Array zurück.
Code
Geil. Übersetzen wir unseren Pseudo-Code in TypeScript:
Lösungsschritte
Meine erste Zeile:
export function sortTheInnerContent(words: string): string {
Dann der Loop:
return words
.split(" ")
.map((word) => {
Wahrscheinlich können wir hier das Ganze mit Method-Chaining lösen, darum setze ich das return
-Keyword direkt an den Anfang.
Wörter die 2
oder weniger Buchstaben haben, brauchen wir nicht verändern:
if (word.length <= 2) return word;
Wir teilen das Wort auf in seine Bestandteile:
const first = word[0];
const middle = word.slice(1, -1);
const last = word.slice(-1);
Jetzt können wir die Mitte sortieren, aufsteigend:
const sortedMiddle = middle
.split("")
.sort((a, b) => b.localeCompare(a))
.join("");
localeCompare()
ist
eine coole Methode, die entsprechend 0
, 1
oder -1
zurückgibt.
Wenn dir localeCompare()
zu unheimlich ist, kannst du alternativ auch z.B. folgendes in deinen Sorter-Function-Body schreiben:
if (a < b) return 1;
if (a > b) return -1;
return 0;
Dann das neue Wort zusammensetzen und ans Array zurückgeben:
return first + sortedMiddle + last;
})
Zum Schluss nicht vergessen das Array wieder zu einem String zusammenzufügen:
.join(" ");
}
Returnen brauchen wir nicht, haben wir ja oben schon gemacht.
Voilá! 💪
Komplettlösung
export function sortTheInnerContent(words: string): string {
return words
.split(" ")
.map((word) => {
if (word.length <= 2) return word;
const first = word[0];
const middle = word.slice(1, -1);
const last = word.slice(-1);
const sortedMiddle = middle
.split("")
.sort((a, b) => b.localCompare(a))
.join("");
return first + sortedMiddle + last;
})
.join(" ");
}