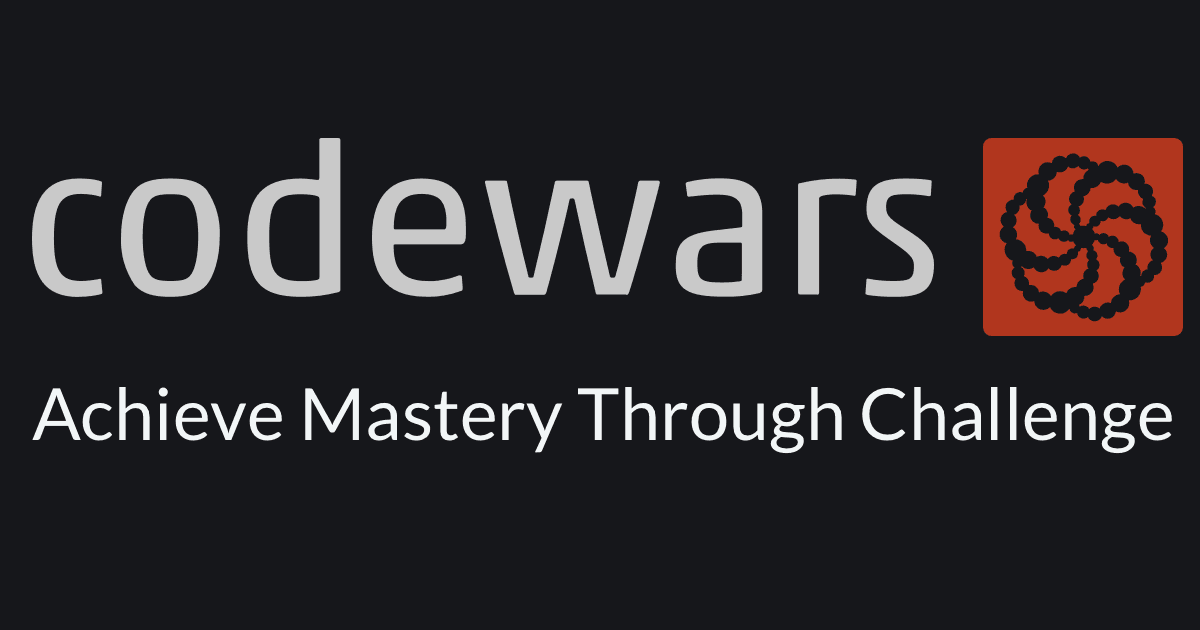
Inhalt
Die Fakten:
Plattform: | codewars.com |
Name: | How Much? |
Level: | 6 kyu |
Sprache: | TypeScript |
Beschreibung:
I always thought that my old friend John was rather richer than he looked, but I never knew exactly how much money he actually had. One day (as I was plying him with questions) he said:
- "Imagine I have between
m
andn
Zloty..." (or did he say Quetzal? I can't remember!) - "If I were to buy 9 cars costing
c
each, I'd only have 1 Zloty (or was it Meticals?) left." - "And if I were to buy 7 boats at
b
each, I'd only have 2 Ringglets (or was it Zloty?) left."
Could you tell me in each possible case:
- how much money
f
he could possibly have ? - the cost
c
of a car? - the cost
b
of a boat?
So, I will have a better idea about his fortune. Note that if m-n
is big enough, you might have a lot of possible answers.
Each answer should be given as ["M: f", "B: b", "C: c"]
and all the answers as [ ["M: f", "B: b", "C: c"], ... ]
. "M" stands for money, "B" for boats, "C" for cars.
Note: m, n, f, b, c
are positive integers, where 0 <= m <= n
or m >= n >= 0
. m
and n
are inclusive.
Examples:
howmuch(1, 100) => [["M: 37", "B: 5", "C: 4"], ["M: 100", "B: 14", "C: 11"]]
howmuch(1000, 1100) => [["M: 1045", "B: 149", "C: 116"]]
howmuch(10000, 9950) => [["M: 9991", "B: 1427", "C: 1110"]]
howmuch(0, 200) => [["M: 37", "B: 5", "C: 4"], ["M: 100", "B: 14", "C: 11"], ["M: 163", "B: 23", "C: 18"]]
Explanation of the results for howmuch(1, 100)
:
- In the first answer his possible fortune is 37:
- so he can buy 7 boats each worth 5:
37 - 7 * 5 = 2
- or he can buy 9 cars worth 4 each:
37 - 9 * 4 = 1
- The second possible answer is 100:
- he can buy 7 boats each worth 14:
100 - 7 * 14 = 2
- or he can buy 9 cars worth 11:
100 - 9 * 11 = 1
Note
See "Sample Tests" to know the format of the return.
Quelle: codewars.com
Lösung
Pseudo-Code
Wie immer gibt's reichlich Varianten, hier ist eine meiner.
Erst die Lösungsschritte in Pseudo-Code. Los geht’s:
Lösungsschritte
Schritt 1
Da mal m
und mal n
größer sein können, müssen wir zuerst herausfinden welches das Größere und welches das Kleinere ist.
Schritt 2
Dann brauchen wir einen Loop.
Schritt 3
Jetzt macht sich mein Mathe-Leistungskurs bezahlt.
Schritt 4
Wenn der Wert aller Boote durch 7
teilbar ist und der Wert aller Autos durch 9
, haben wir eine mögliche Konstellation.
Schritt 5
In diesem Fall hängen wir diese Konstellation an ein Array an.
Code
Geil. Übersetzen wir unseren Pseudo-Code in TypeScript:
Lösungsschritte
Meine erste Zeile:
export function howMuch(m: number, n: number): string[][] {
Als Erstes also ermitteln wir, welches die große und welches die kleine Zahl ist:
const [min, max] = [m, n].sort((a, b) => a - b);
const result: string[][] = [];
Bei dieser Gelegenheit initialisiere ich mir auch gleich das Ergebnis-Array für die möglichen Konstellationen.
Jetzt können wir fröhlich loopen:
for (let i = min; i <= max; i++) {
Der Lesbarkeit halber speichere ich mir den Wert aller Boote und Autos jeweils in einer Variablen ab (optional):
const allBoatsValue = i - 2;
const allCarsValue = i - 1;
Jetzt können wir prüfen, ob der Wert aller Boote durch 7
und der Wert aller Autos durch 9
teilbar ist:
if (allBoatsValue % 7 === 0 && allCarsValue % 9 === 0) {
Wenn ja, können wir diese mögliche Konstellation ans Ergebnis-Array anhängen:
const money = i;
const costBoat = allBoatsValue / 7;
const costCar = allCarsValue / 9;
result.push([`M: ${money}`, `B: ${costBoat}`, `C: ${costCar}`]);
}
}
Der Lesbarkeit halber speichere ich mir die Werte vorher noch mal jeweils in einer Variablen ab (optional).
Nach dem Loop nur noch unsere Ergebnisse zurückgeben:
return result;
}
Voilá! 💪
Komplettlösung
export function howMuch(m: number, n: number): string[][] {
const [min, max] = [m, n].sort((a, b) => a - b);
const result: string[][] = [];
for (let i = min; i <= max; i++) {
const allBoatsValue = i - 2;
const allCarsValue = i - 1;
if (allBoatsValue % 7 === 0 && allCarsValue % 9 === 0) {
const money = i;
const costBoat = allBoatsValue / 7;
const costCar = allCarsValue / 9;
result.push([`M: ${money}`, `B: ${costBoat}`, `C: ${costCar}`]);
}
}
return result;
}