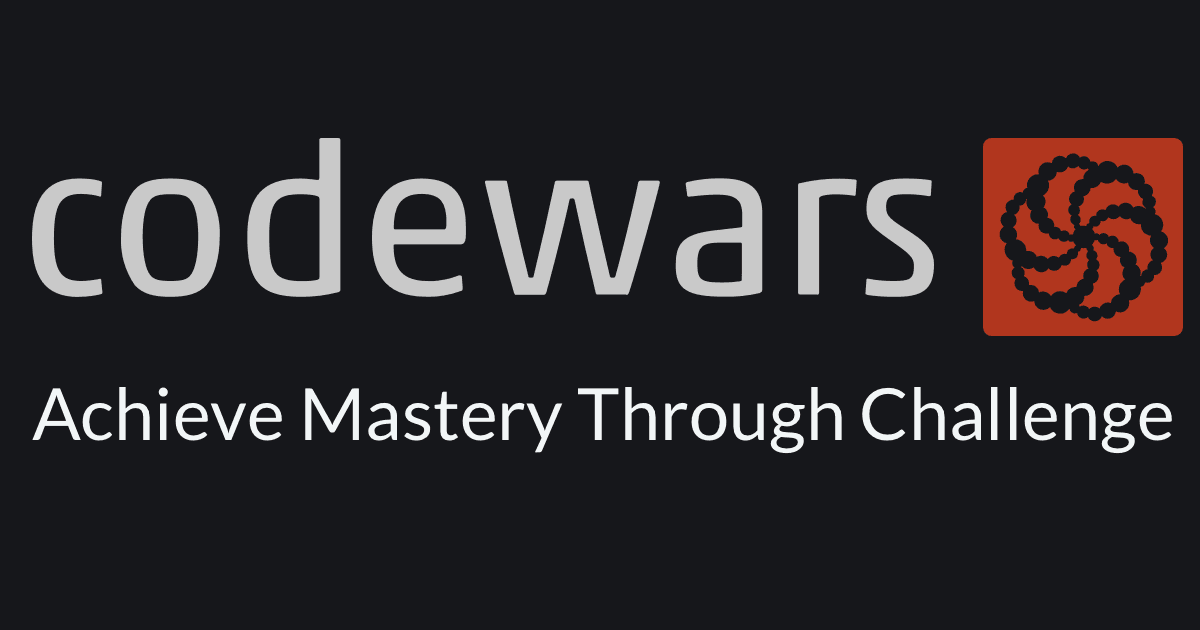
Inhalt
Die Fakten:
Plattform: | codewars.com |
Name: | Hëävÿ Mëtäl Ümläüts |
Level: | 7 kyu |
Sprache: | TypeScript |
Beschreibung:
Your retro heavy metal band, VÄxën, originally started as kind of a joke, just because why would anyone want to use the crappy foosball table in your startup's game room when they could be rocking out at top volume in there instead? Yes, a joke, but now all the top tech companies are paying you top dollar to play at their conferences and big product-release events. And just as how in the early days of the Internet companies were naming everything "i"-this and "e"-that, now that VÄxënmänïä has conquered the tech world, any company that doesn't use Hëävÿ Mëtäl Ümläüts in ëvëry pössïblë pläcë is looking hopelessly behind the times.
Well, with great power chords there must also come great responsibility! You need to help these companies out by writing a function that will guarantee that their web sites and ads and everything else will RÖCK ÄS MÜCH ÄS PÖSSÏBLË! Just think about it -- with the licensing fees you'll be getting from Gööglë, Fäcëböök, Äpplë, and Ämäzön alone, you'll probably be able to end world hunger, make college and Marshall stacks free to all, and invent self-driving bumper cars. Sö lët's gët röckïng and röllïng! Pëdal tö thë MËTÄL!
Here's a little cheatsheet that will help you write your function to replace the so-last-year letters a-e-i-o-u-and-sometimes-y with the following totally rocking letters:
A = Ä = \u00c4 E = Ë = \u00cb I = Ï = \u00cf
O = Ö = \u00d6 U = Ü = \u00dc Y = Ÿ = \u0178
a = ä = \u00e4 e = ë = \u00eb i = ï = \u00ef
o = ö = \u00f6 u = ü = \u00fc y = ÿ = \u00ff
Quelle: codewars.com
Lösung
Pseudo-Code
Wie immer gibt's reichlich Varianten, hier ist eine meiner.
Erst die Lösungsschritte in Pseudo-Code. Los geht’s:
Lösungsschritte
Schritt 1
Als Erstes brauchen wir einen Schlüssel, in dem festgelegt ist, welcher Buchstabe mit welchem ersetzt werden soll.
Schritt 2
Dann können wir durch den Input Text loopen.
Schritt 3
Wenn es eine Entsprechung in unserem Schlüssel gibt, soll der jeweils aktuelle Buchstabe damit ersetzt werden, sonst nicht.
Code
Geil. Übersetzen wir unseren Pseudo-Code in TypeScript:
Lösungsschritte
Meine erste Zeile:
export function heavyMetalUmlauts(boringText: string): string {
Jetzt müssen wir unsere Daten so hinterlegen, dass wir sie nachschlagen können. Z.B. in einem Objekt:
const map: { [key: string]: string } = {
A: "Ä",
E: "Ë",
I: "Ï",
O: "Ö",
U: "Ü",
Y: "Ÿ",
a: "ä",
e: "ë",
i: "ï",
o: "ö",
u: "ü",
y: "ÿ",
};
Das war schon mal die halbe Miete und hat mich am meisten Zeit gekostet... TypeScript
sagen wir hier, dass key
und value
jeweils Strings sind. Ich nenne mein Schlüssel-Objekt map
.
Jetzt nur noch durch den String loopen und für jeden Buchstaben seine Übersetzung zurückgeben (wenn es eine gibt):
return [...boringText].map((char) => (map[char] ? map[char] : char)).join("");
}
Hier spreaden wir unseren String in ein Array damit wir .map()
anwenden können. Dann prüfen wir (mit einem Ternary
für jedes Element char
, ob es in unserem map
-Objekt existiert.
Wenn ja, geben wir den zugehörigen Wert zurück. Wenn nicht, geben wir einfach das Element unverändert zurück.
Zum Schluss joinen
wir das Array wieder zu einem String.
Voilá! 💪
Komplettlösung
export function heavyMetalUmlauts(boringText: string): string {
const map: { [key: string]: string } = {
A: "Ä",
E: "Ë",
I: "Ï",
O: "Ö",
U: "Ü",
Y: "Ÿ",
a: "ä",
e: "ë",
i: "ï",
o: "ö",
u: "ü",
y: "ÿ",
};
return [...boringText].map((char) => (map[char] ? map[char] : char)).join("");
}