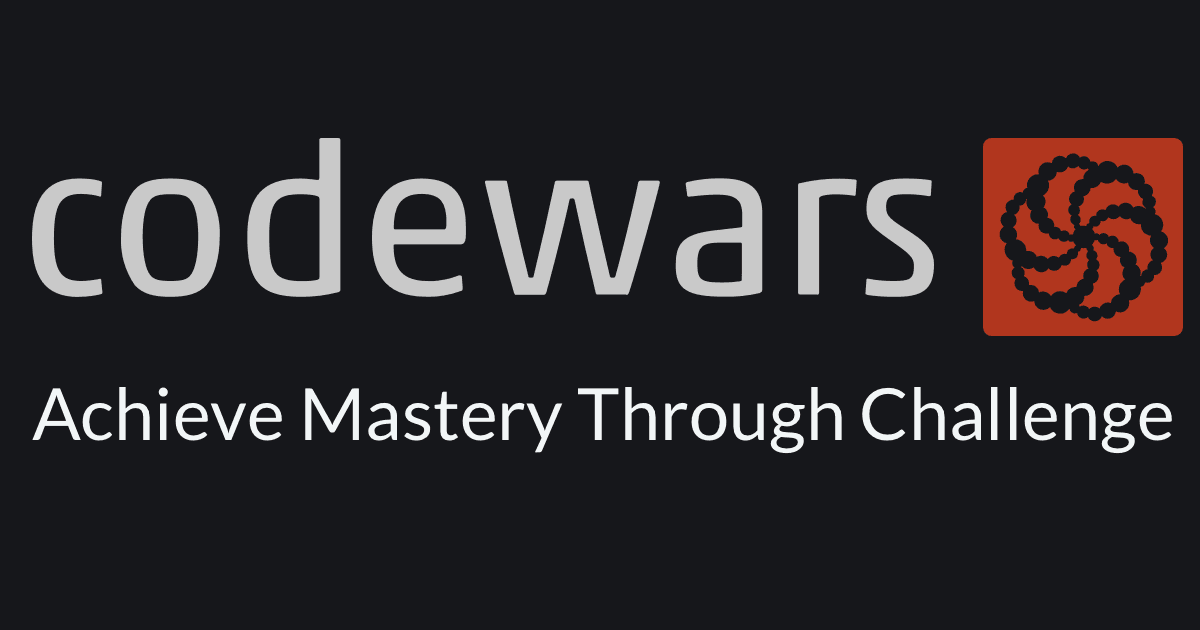
Inhalt
Die Fakten:
Plattform: | codewars.com |
Name: | Fruit Machine |
Level: | 6 kyu |
Sprache: | TypeScript |
Beschreibung:
Introduction
Slot machine (American English), informally fruit machine (British English), puggy (Scottish English slang), the slots (Canadian and American English), poker machine (or pokies in slang) (Australian English and New Zealand English) or simply slot (American English), is a casino gambling machine with three or more reels which spin when a button is pushed. Slot machines are also known as one-armed bandits because they were originally operated by one lever on the side of the machine as distinct from a button on the front panel, and because of their ability to leave the player in debt and impoverished. Many modern machines are still equipped with a legacy lever in addition to the button. (Source Wikipedia)

Task
You will be given three reels of different images and told at which index the reels stop. From this information your job is to return the score of the resulted reels.
Rules
1. There are always exactly three reels
2. Each reel has 10 different items.
3. The three reel inputs may be different.
4. The spin array represents the index of where the reels finish.
5. The three spin inputs may be different
6. Three of the same is worth more than two of the same
7. Two of the same plus one "Wild" is double the score.
8. No matching items returns 0.
Scoring
Returns
Return an integer of the score.
Example
Initialise
reel1 = ["Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"]
reel2 = ["Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"]
reel3 = ["Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"]
spin = [5,5,5]
result = fruit([reel1,reel2,reel3],spin)
reel1 = ["Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"]
reel2 = ["Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"]
reel3 = ["Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"]
spin = [5,5,5]
result = fruit([reel1,reel2,reel3],spin)
reel1 = ["Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"];
reel2 = ["Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"];
reel3 = ["Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"];
spin = [5,5,5];
result = fruit([reel1,reel2,reel3],spin);
Kata kata = new Kata();
string[] reel1 = new string[] { "Wild", "Star", "Bell", "Shell", "Seven", "Cherry", "Bar", "King", "Queen", "Jack" };
string[] reel2 = new string[] { "Wild", "Star", "Bell", "Shell", "Seven", "Cherry", "Bar", "King", "Queen", "Jack" };
string[] reel3 = new string[] { "Wild", "Star", "Bell", "Shell", "Seven", "Cherry", "Bar", "King", "Queen", "Jack" };
List reels = new List { reel1, reel2, reel3 };
int[] spins = new int[] { 5, 5, 5 };
int result = kata.fruit(reels, spins);
reel1 = ["Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"]
reel2 = ["Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"]
reel3 = ["Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"]
spin = [5,5,5]
result = fruit [reel1,reel2,reel3] spin
reel1 = {"Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"}
reel2 = {"Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"}
reel3 = {"Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"}
spin = {5,5,5}
result = fruit({reel1, reel2, reel3}, spin)
reel1 = {"Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"}
reel2 = {"Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"}
reel3 = {"Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"}
spin = {5,5,5}
result = fruit({reel1, reel2, reel3}, spin)
reel1 = {"Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"}
reel2 = {"Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"}
reel3 = {"Wild","Star","Bell","Shell","Seven","Cherry","Bar","King","Queen","Jack"}
spin = {5,5,5}
result = fruit({reel1, reel2, reel3}, spin)
Scoring
reel1!!5 == "Cherry"
reel2!!5 == "Cherry"
reel3!!5 == "Cherry"
Cherry + Cherry + Cherry == 50
reel1[5] == "Cherry"
reel2[5] == "Cherry"
reel3[5] == "Cherry"
Cherry + Cherry + Cherry == 50
reel1[5] == "Cherry"
reel2[5] == "Cherry"
reel3[5] == "Cherry"
Cherry + Cherry + Cherry == 50
reel1[5] == "Cherry"
reel2[5] == "Cherry"
reel3[5] == "Cherry"
Cherry + Cherry + Cherry == 50
reel1[5] == "Cherry"
reel2[5] == "Cherry"
reel3[5] == "Cherry"
Cherry + Cherry + Cherry == 50
reel1[5] == "Cherry"
reel2[5] == "Cherry"
reel3[5] == "Cherry"
Cherry + Cherry + Cherry == 50
reel1[5] == "Cherry"
reel2[5] == "Cherry"
reel3[5] == "Cherry"
Cherry + Cherry + Cherry == 50
reel1[5] == "Cherry"
reel2[5] == "Cherry"
reel3[5] == "Cherry"
Cherry + Cherry + Cherry == 50
reel1[5] == "Cherry"
reel2[5] == "Cherry"
reel3[5] == "Cherry"
Cherry + Cherry + Cherry == 50
Return
result == 50
Good luck and enjoy!
Kata Series
If you enjoyed this, then please try one of my other Katas. Any feedback, translations and grading of beta Katas are greatly appreciated. Thank you.
Quelle: codewars.com
Lösung
Pseudo-Code
Wie immer gibt's reichlich Varianten, hier ist eine meiner.
Erst die Lösungsschritte in Pseudo-Code. Los geht’s:
Lösungsschritte
Schritt 1
Als Erstes erstellen wir uns ein Array mit den Symbolen.
Schritt 2
Die entsprechenden Punkte können wir aus dem Index ermitteln.
Schritt 3
Dann loopen wir durch die 3
Spins und ermitteln für jeden Spin das entsprechende Symbol.
Schritt 4
Danach können wir die doppelten Symbole entfernen und kucken wie viele übrig bleiben.
Schritt 5
Wenn 1
übrig bleibt, hatten wir 3
gleiche und können die Punkte mal 10
nehmen.
Schritt 6
Wenn 2
übrig bleiben, müssen wir noch schauen, ob ein "Wild"
dabei ist.
Schritt 7
Wenn ja, Punkte mal 2
.
Schritt 8
Wenn nicht, einfache Punkte.
Schritt 9
Ansonsten geben wir 0
Punkte zurück.
Code
Geil. Übersetzen wir unseren Pseudo-Code in TypeScript:
Lösungsschritte
Meine erste Zeile:
export function fruit(reels: string[][], spins: number[]): number {
Dann das Array:
const items = "_,Jack,Queen,King,Bar,Cherry,Seven,Shell,Bell,Star,Wild".split(
",",
);
Da ich ein bisschen faul bin und keinen Bock hab die ganzen Anführungszeichen zu tippen, erstelle ich einfach einen String, den ich direkt in ein Array umwandle. 🤤
Außerdem setze ich ein Dummy-Element an den Anfang, damit das erste richtige Element den Index 1
hat.
Jetzt der Loop durch die Spins um die Symbole zu ermitteln:
const sortedResults = spins.map((spin, i) => reels[i][spin]).sort();
Außerdem sortiere ich hier das Ergebnis direkt. Das wird uns gleich hilfreich sein...
Jetzt können wir schon mal die Punkte für 2
gleiche Symbole ermitteln:
const middle = sortedResults[1];
const points = items.indexOf(middle);
Wenn wir ein doppeltes Symbol dabei haben, dann ist es nach dem Sortieren auf jeden Fall in der Mitte. Die Punkte für dieses Symbol entnehmen wir dem Index im Array.
Dann können wir die doppelten Symbole entfernen:
const differentItems = [...new Set(sortedResults)];
Mit Set
entfernen wir einfach und effizient alle doppelten Elemente. Dann erstellen wir daraus sofort wieder ein Array.
Cool! Jetzt können wir schauen wie viele Elemente übrig geblieben sind und dementsprechend die Punkte berechnen.
Wenn wir nur noch 1
Symbol übrig haben, waren es 3
gleiche. Wir können die Punkte mit 10
multiplizieren:
if (differentItems.length === 1) return points * 10;
Wenn wir noch 2
Symbole übrig haben, war ein Päarchen dabei. In diesem Fall müssen wir noch checken, ob genau ein "Wild"
in den ursprünglichen sortierten Ergebnissen ist:
if (differentItems.length === 2) {
if (sortedResults.filter((result) => result === "Wild").length === 1) {
Wenn ja, multiplizieren wir unsere Punkte mit 2
, ansonsten lassen wir sie, wie sie sind:
return points * 2;
}
return points;
}
Ansonsten - also wenn wir immer noch 3
verschiedene Symbole haben - geben wir 0
Punkte zurück:
return 0;
}
Voilá! 💪
Komplettlösung
export function fruit(reels: string[][], spins: number[]): number {
const items = "_,Jack,Queen,King,Bar,Cherry,Seven,Shell,Bell,Star,Wild".split(
",",
);
const sortedResults = spins.map((spin, i) => reels[i][spin]).sort();
const middle = sortedResults[1];
const points = items.indexOf(middle);
const differentItems = [...new Set(sortedResults)];
if (differentItems.length === 1) return points * 10;
if (differentItems.length === 2) {
if (sortedResults.filter((result) => result === "Wild").length === 1) {
return points * 2;
}
return points;
}
return 0;
}