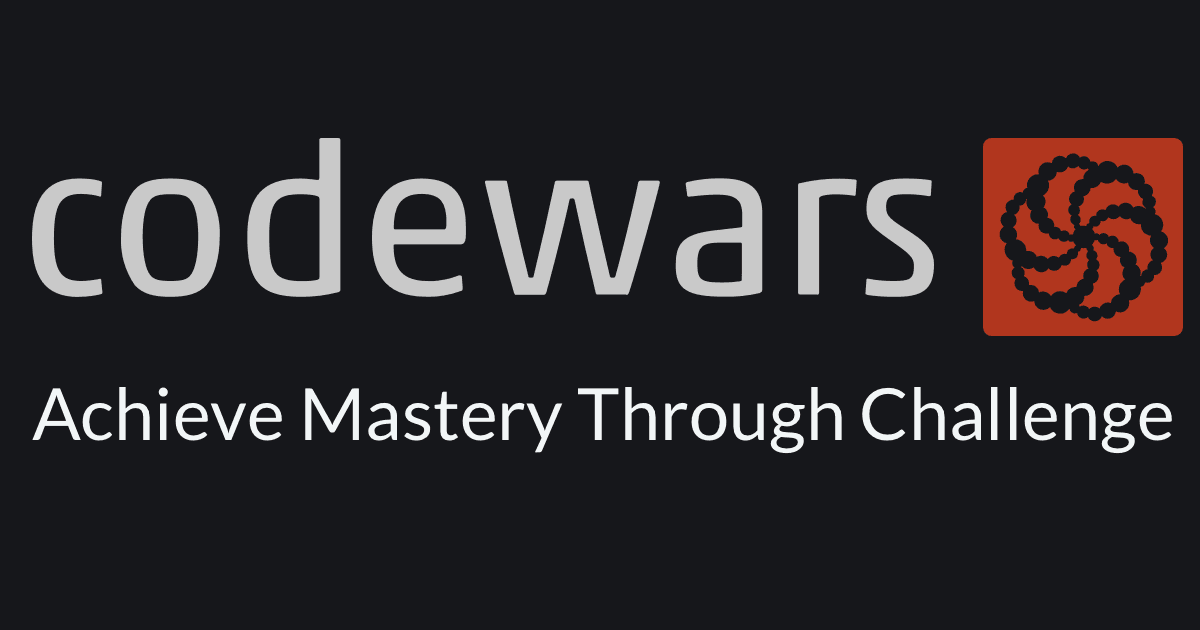
Inhalt
Die Fakten:
Plattform: | codewars.com |
Name: | For Twins: 2. Math operations |
Level: | 8 kyu |
Sprache: | TypeScript |
Beschreibung:
Task:
A magician in the subway showed you a trick, he put an ice brick in a bottle to impress you. The brick's length and width are equal, forming a square; its height may be different. Just for fun and also to impress the magician and people around, you decided to calculate the brick's volume. Write a function iceBrickVolume
that will accept these parameters:
radius
- bottle's radius (always > 0);bottleLength
- total bottle length (always > 0);rimLength
- length from bottle top to brick (always <bottleLength
);
And return volume of ice brick that magician managed to put into a bottle.
Note:
All inputs are integers. Assume no irregularities to the cuboid brick. You may assume the bottle is shaped like a cylinder. The brick cannot fit inside the rim. The ice brick placed into the bottle is the largest possible cuboid that could actually fit inside the inner volume.
Example 1:
radius = 1
bottle_length = 10
rim_length = 2
volume = 16
Example 2:
radius = 5
bottle_length = 30
rim_length = 7
volume = 1150
Quelle: codewars.com
Lösung
Pseudo-Code
Wie immer gibt's reichlich Varianten, hier ist eine meiner.
Erst die Lösungsschritte in Pseudo-Code. Los geht’s:
Lösungsschritte
Schritt 1
Als Erstes müssen wir rauskriegen was die Länge bzw. Breite der Grundfläche des Würfels ist. Dafür machen wir uns am besten eine Zeichnung.
Schritt 2
Dann können wir die Höhe des Würfels berechnen.
Schritt 3
Wenn wir alle 3
Seiten haben, können wir das Volumen berechnen.
Code
Geil. Übersetzen wir unseren Pseudo-Code in TypeScript:
Lösungsschritte
Meine erste Zeile:
export const iceBrickVolume = (radius: number, bottleLength: number, rimLength: number): number => {
Dann berechnen wir also die Länge bzw. Breite der Grundfläche des Würfels:
const brickLength = Math.sqrt(radius ** 2 + radius ** 2);
Wir wissen, dass der größtmögliche Würfel in der Flasche die Flaschenwand an 4
Punkten berührt. Und wir haben den Radius der Flasche.
Wenn wir jetzt 2
Geraden von der Mitte aus mit jeweils der Länge des Radius im rechten Winkel ziehen - etwa so: |_
, erhalten wir 2
Berührpunkte des Würfels mit dem Flaschenrand.
Wenn wir diese beiden Punkte miteinander verbinden, erhalten wir die Länge einer der 4
Seiten der Grundfläche des Würfels.
Um die Länge der Seite gegenüber des rechten Winkels in einem Dreieck zu berechnen, können wir den Satz des Pythagoras verwenden.
Satz des Pythagoras:
nach c
auflösen, also Wurzel ziehen:
Übersetzt für unseren Fall:
brickLength = Math.sqrt(radius ** 2 + radius ** 2);
Jetzt berechnen wir noch schnell die Höhe des Würfels:
const brickHeight = bottleLength - rimLength;
Und zum Schluss nur noch das Volumen berechnen und zurückgeben:
return Math.round(brickLength * brickLength * brickHeight);
};
Voilá! 💪
Komplettlösung
export const iceBrickVolume = (
radius: number,
bottleLength: number,
rimLength: number,
): number => {
const brickLength = Math.sqrt(radius ** 2 + radius ** 2); // c^2 = a^2 + b^2
const brickHeight = bottleLength - rimLength;
return Math.round(brickLength * brickLength * brickHeight);
};