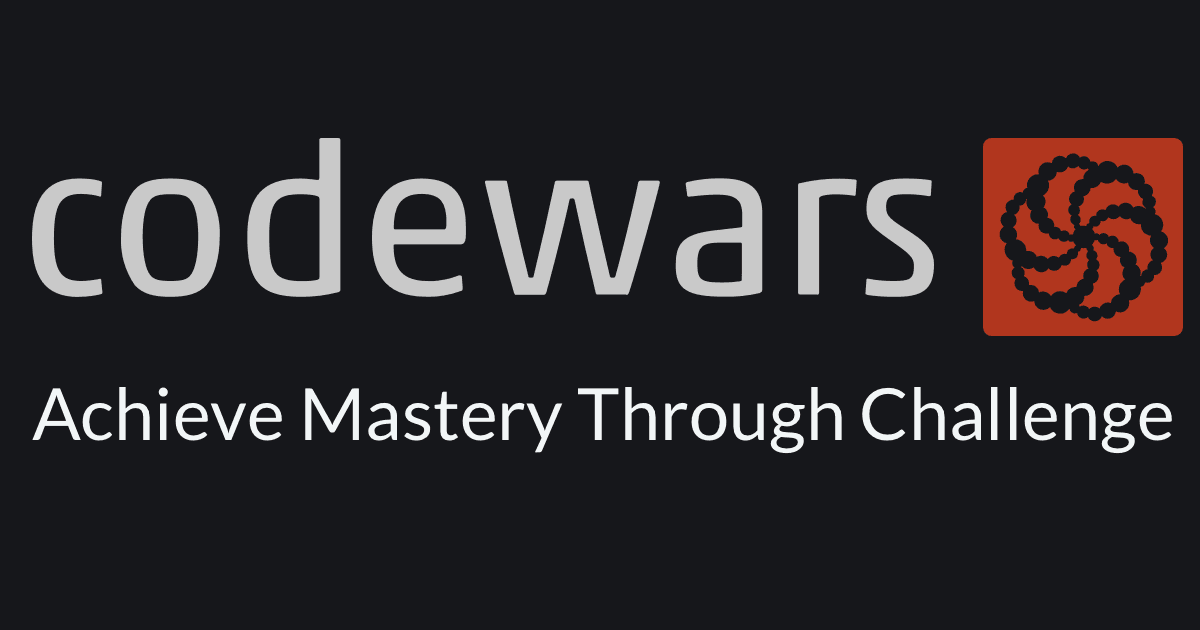
Inhalt
Die Fakten:
Plattform: | codewars.com |
Name: | Binary to Text (ASCII) Conversion |
Level: | 6 kyu |
Sprache: | TypeScript |
Beschreibung:
Write a function that takes in a binary string and returns the equivalent decoded text (the text is ASCII encoded).
Each 8 bits on the binary string represent 1 character on the ASCII table.
The input string will always be a valid binary string.
Characters can be in the range from "00000000" to "11111111" (inclusive)
Note: In the case of an empty binary string your function should return an empty string.
Quelle: codewars.com
Lösung
Pseudo-Code
Wie immer gibt's reichlich Varianten, hier ist eine meiner.
Erst die Lösungsschritte in Pseudo-Code. Los geht’s:
Lösungsschritte
Schritt 1
Wir loopen durch das Input-Binary.
Schritt 2
In jeder Iteration schnappen wir uns die nächsten 8
Zeichen.
Schritt 3
Diese 8
Bits wandeln wir in ihre dezimale Entsprechung um.
Schritt 4
Und speichern das Ergebnis in einer Variablen.
Code
Geil. Übersetzen wir unseren Pseudo-Code in TypeScript:
Lösungsschritte
Meine erste Zeile:
export function binaryToString(binary: string): string {
Als Erstes der Loop:
for (let i = 0; i < binary.length; i += 8) {
i
erhöhen wir hier bei jeder Iteration um 8
.
Jetzt schnappen wir uns das aktuelle 8
-Bit Stück und speichern es in einer Variablen zwischen:
const curr8Bits = binary.slice(i, i + 8);
Dann können wir das aktuelle 8
-Bit Stück in eine Zahl, den ASCII-Code umwandeln:
const currCharCode = parseInt(curr8Bits, 2);
Und jetzt den aktuellen ASCII-Code in ein Zeichen/Buchstaben umwandeln und zu einer Ergebnis-Variablen hinzufügen:
result += String.fromCharCode(currCharCode);
}
Die Variable muss natürlich noch oben initialisiert werden.
let result = "";
Nach dem Loop nur noch das Ergebnis zurückgeben:
return result;
}
Voilá! 💪
Komplettlösung
export function binaryToString(binary: string): string {
let result = "";
for (let i = 0; i < binary.length; i += 8) {
const curr8Bits = binary.slice(i, i + 8);
const currCharCode = parseInt(curr8Bits, 2);
result += String.fromCharCode(currCharCode);
}
return result;
}